Over the course of many client engagements, we at TrackIt noticed that AWS Cognito login and authentication flows were being used on a recurring basis. To streamline future solutions development, we decided to build a boilerplate/integration framework that would allow clients to kickstart their deployments by quickly interfacing their applications with Cognito.
“During my multiple engagements with clients, I found myself having to do the same technical work over and over again with Cognito. I felt that the natural solution would be to build a tool that makes our development efforts easier.”
– Jéremy, Front End Software Engineer, TrackIt
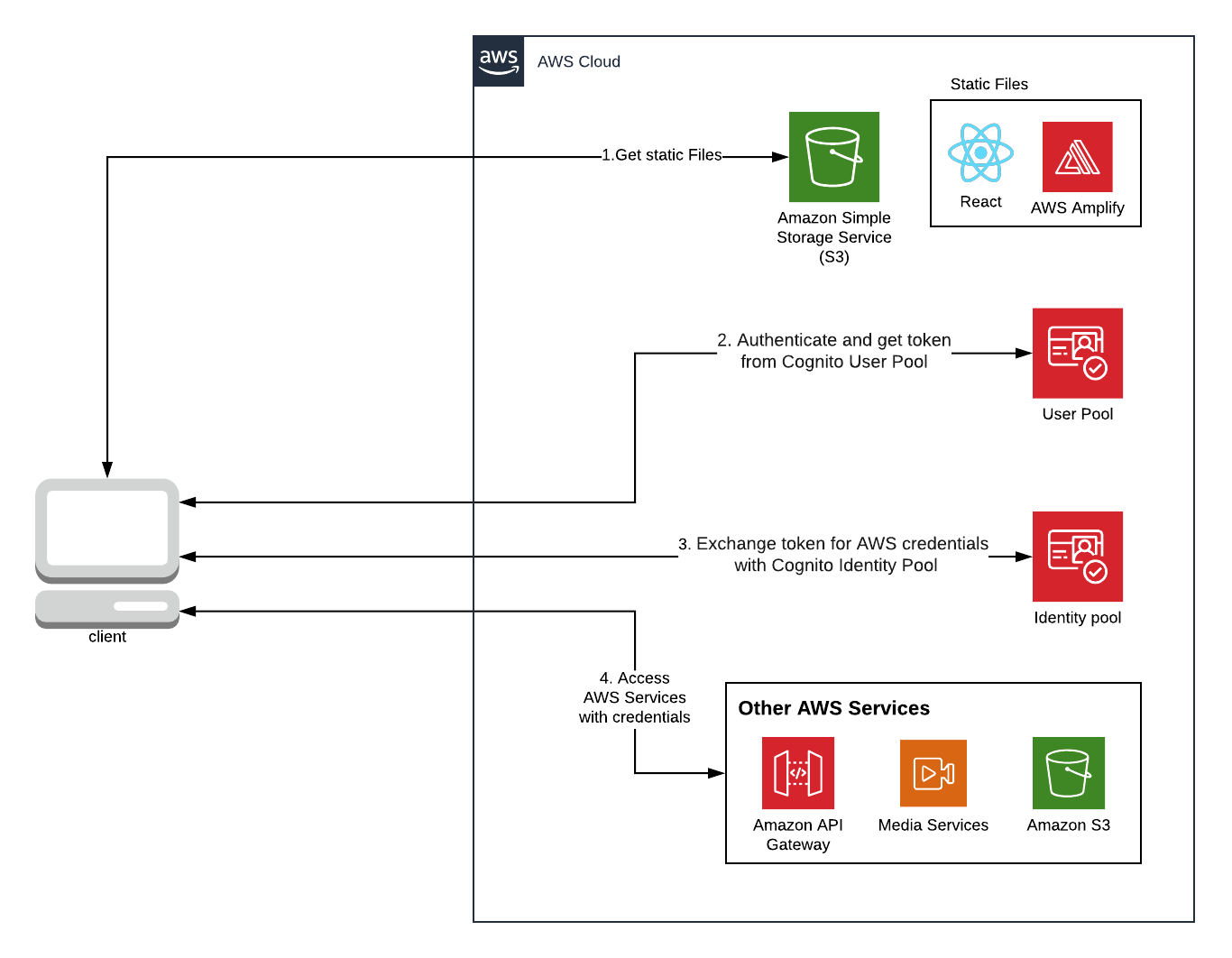
Contents
Cognito
Amazon Cognito provides authentication, authorization, and user management for web and mobile apps. Amazon Cognito has two main components: user pools and identity pools. User pools are user directories that provide sign up and sign in options for app users. Identity pools on the other hand help grant users access to other AWS services. Identity pools and user pools can be used separately or together.
Amplify
AWS Amplify helps build secure and scalable mobile and web applications. Amplify simplifies the process of authenticating users, securely storing data and user metadata, authorizing selective access to data, integrating machine learning, analyzing application metrics, and executing server-side code.
Using Amplify with Cognito is efficient because we can delegate tasks such as token refreshing and authentication to Amplify. Amplify also offers a CLI that automatically configures your project (for Yarn users who are encountering problems with dependencies, take a look at this issue: https://github.com/aws-amplify/amplify-cli/issues/2746)
Amplify provides multiple packages (aws-amplify-react / @aws-amplify/ui-react) which include built-in components for authentication (simple components, higher-order components a.k.a. HOCs, etc.). Amplify also allows customization of fields and appearance.
Note: If you are using custom signUpFields to customize the username field, then you need to make sure that either the label of that field is the same value you set in usernameAttributes or the key of the field is username.
How to Implement Amplify and Cognito without the Amplify CLI
Create a user pool and an identity pool on AWS Cognito
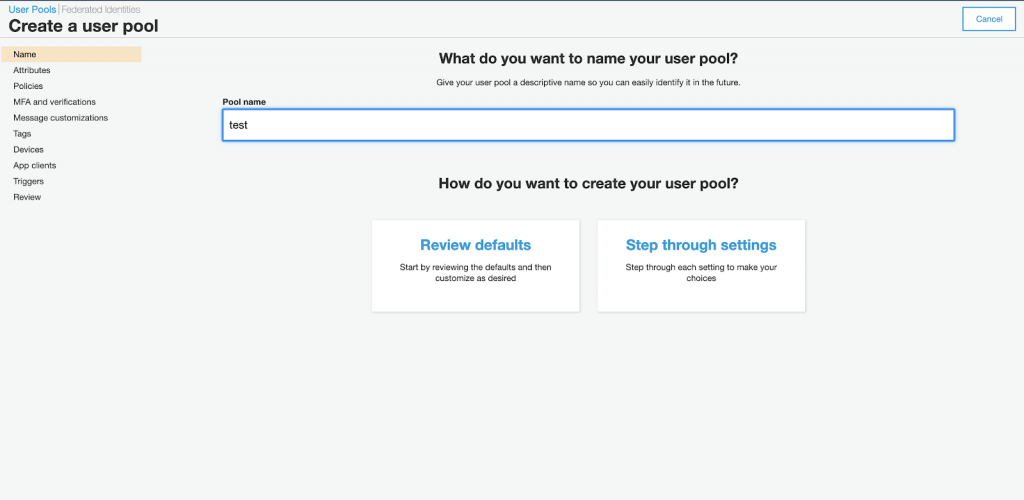
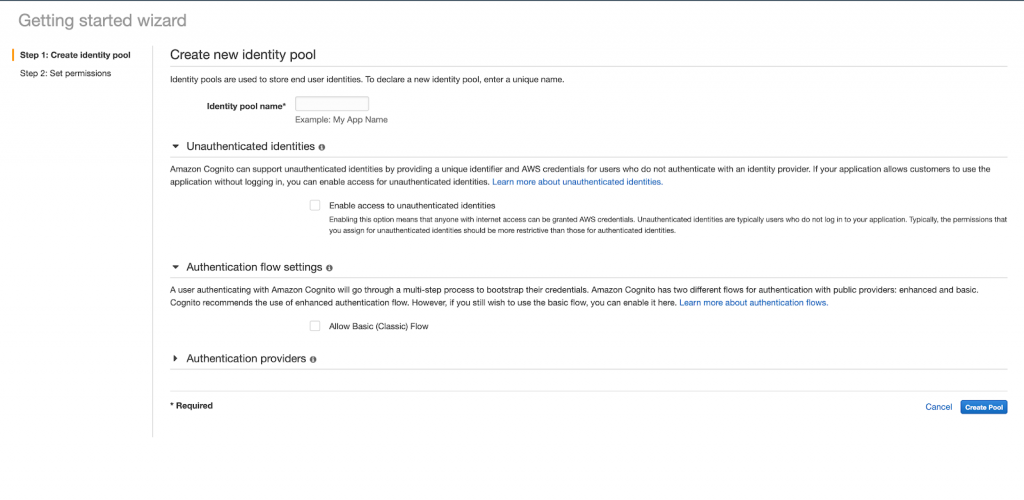
Create an App client
While creating the App client, make sure the “Generate client secret” box is unchecked. JavaScript SDK doesn’t support apps that have a client secret.
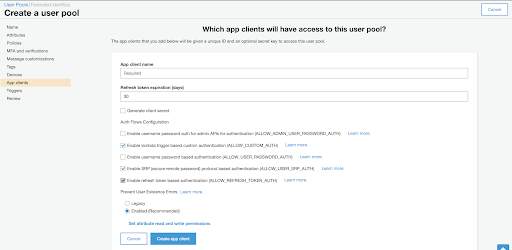
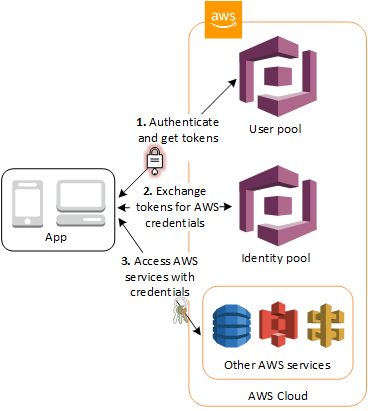
Create a .env file based on example.env with the correct IDs
# Amplify Auth config
REACT_APP_IDENTITY_POOL_ID=""
REACT_APP_REGION=""
REACT_APP_USER_POOL_ID=""
REACT_APP_USER_POOL_WEBCLIENT_ID=""
REACT_APP_API=""
The variables mentioned above are used inside an AmplifyConfig object in shared/amplify.config.ts
The Amplify configuration is based on the instructions mentioned in the following guide: https://aws-amplify.github.io/docs/js/authentication#manual-setup
export const amplifyConfig: AmplifyConfig = {
Auth: {
identityPoolId: process.env.REACT_APP_IDENTITY_POOL_ID,
region: process.env.REACT_APP_REGION,
userPoolId: process.env.REACT_APP_USER_POOL_ID,
userPoolWebClientId: process.env.REACT_APP_USER_POOL_WEBCLIENT_ID,
},
language: “us”,
};
We created a singleton class extending the Amplify class. This class contains an init() method that takes an AmplifyConfig object as a parameter.
This AmplifyConfig is passed to the static configure() method of Amplify that initializes our app with our configuration file.
You can customize the configuration file inside src/shared/amplify.config.ts
We just have to call Config.getInstance().init() at the root of the project (src/index.tsx).
Amplify will handle the register/login process based on our signUpFields configuration (in shared/amplify.config.ts) and display the wrapped components if the authState changes to signedIn.
Depending on your configuration you can add more services (S3 for example). Amplify will apply these settings and will use your identity pool to grant you access to these Amazon services.
Authentication Flow Visuals
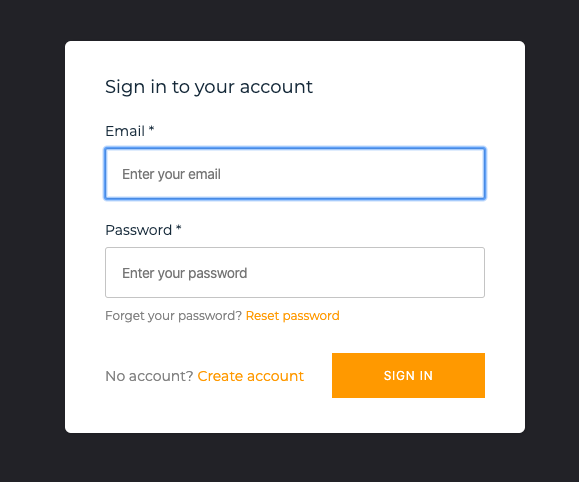
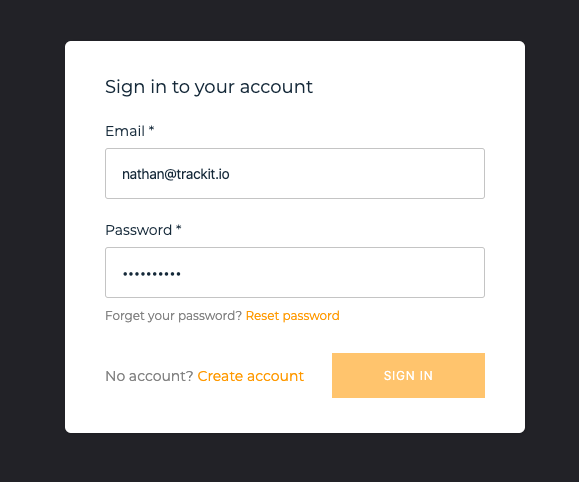
UI Customization
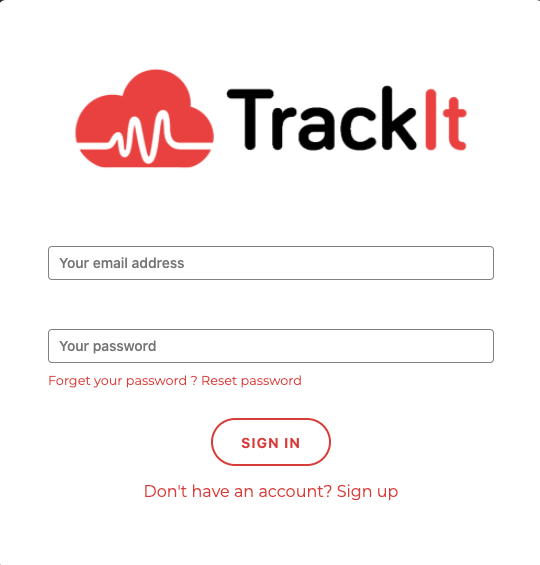
To customize the components and reuse the render logic along with the built-in methods, we need to extend the original components provided by Amplify using React inheritance.
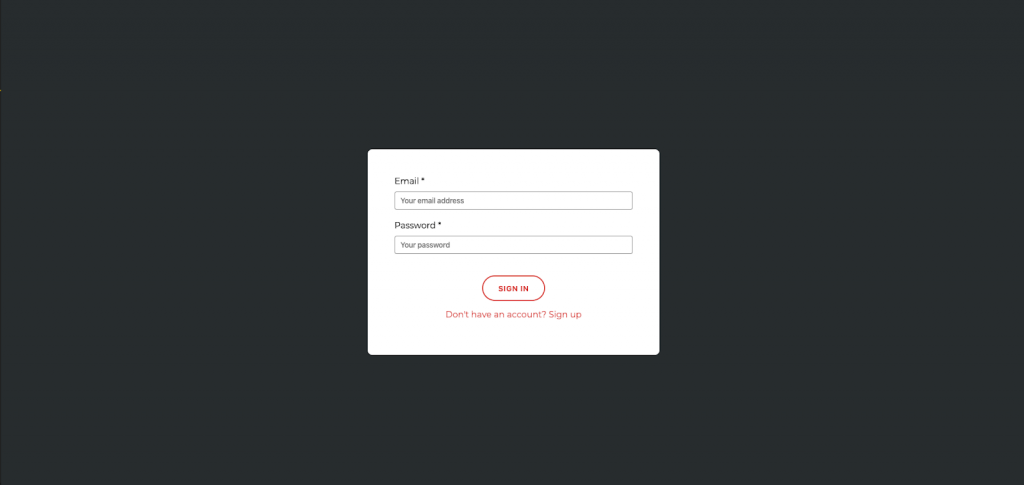
Original components
Here’s an example of how you can customize your UI’s logo using React inheritance.
To add your logo, you need to display a logo on the Auth components. Often, this logo comes from a URL and thus you need to have a logoUrl property of type string. Since you cannot add new properties, you have to pass a component as a child of our component as a workaround.
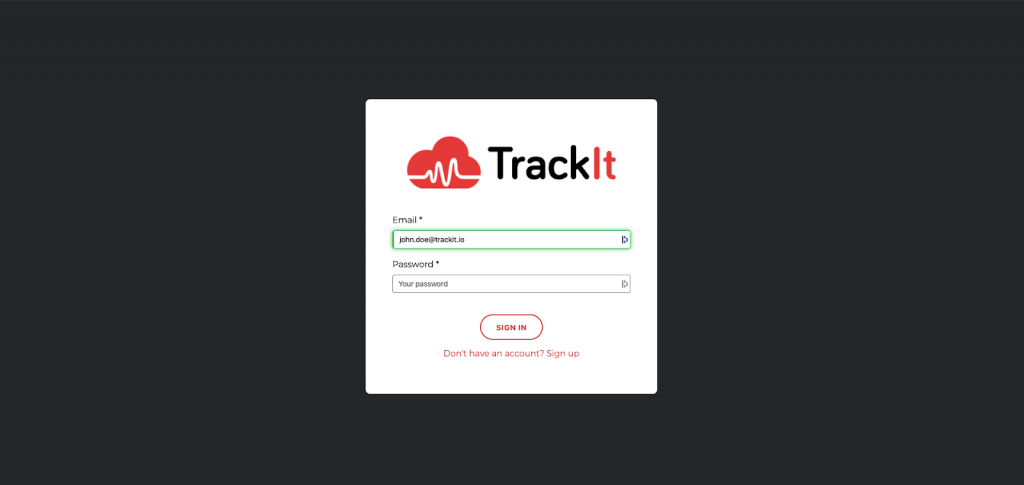
The same process can be used for other UI components as well.
Now your boilerplate is ready to use inside your new or existing projects that require an authentication flow with, or without, custom components.
Here is a link that provides you with the necessary details to customize all your components:
Sample Use Case: Amplify Video
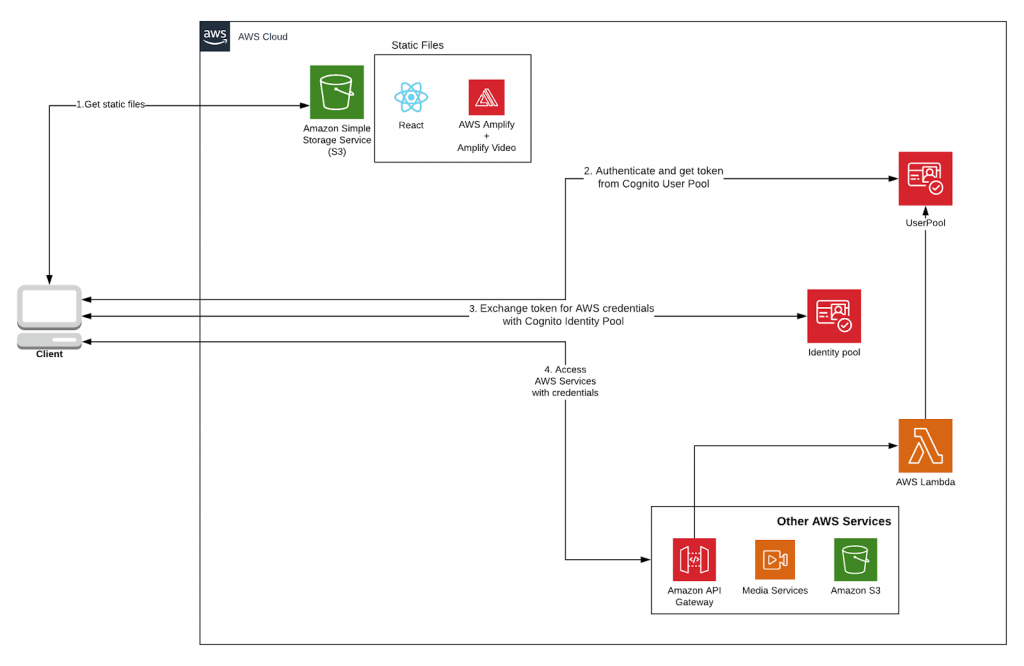
Here’s a sample use case of how to create a React app with role-based user access controls using Cognito groups to allow specific users to watch a live stream.
We added the following features to our original boilerplate:
- Amplify-video – that provides the live stream
- Admin queries – to manage users
- Cognito groups – for role-based user access control using
What follows is a detailed walk through that demonstrates how to implement this live streaming solution with user authentication & management.
Run the following command in the repository:git checkout example/aws-cognito-amplify-video
yarn
ornpm i
You also have to install Amplify-CLIyarn global add @aws-amplify/cli
ornpm i -g @aws-amplify/cli
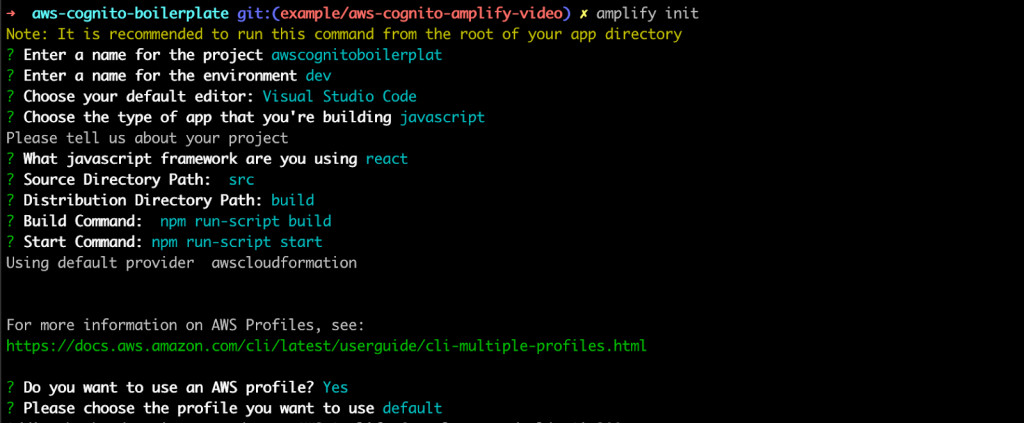
Then run:amplify init
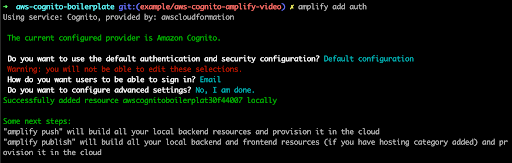
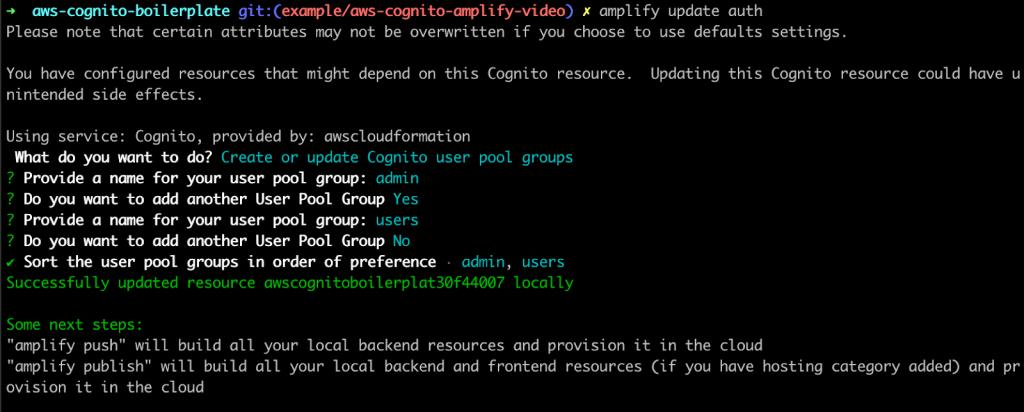
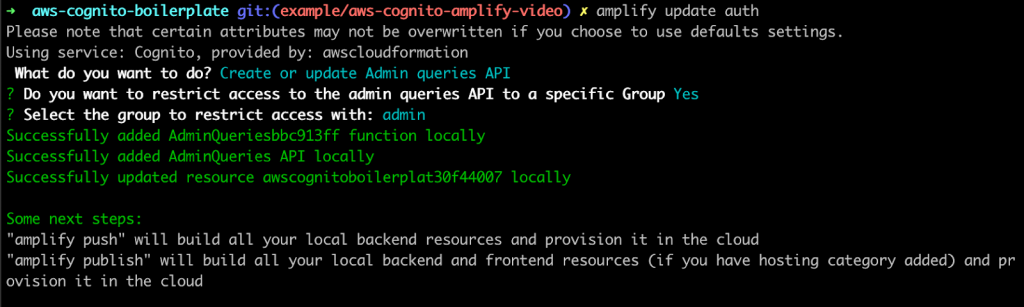
Next, add auth and admin queries :amplify add auth
amplify update auth
amplify push
Copy the .sample.env file and fill REACT_APP_API=”AdminQueries”
Next, add amplify-videoyarn global add amplify-category-video
ornpm i -g amplify-category-video
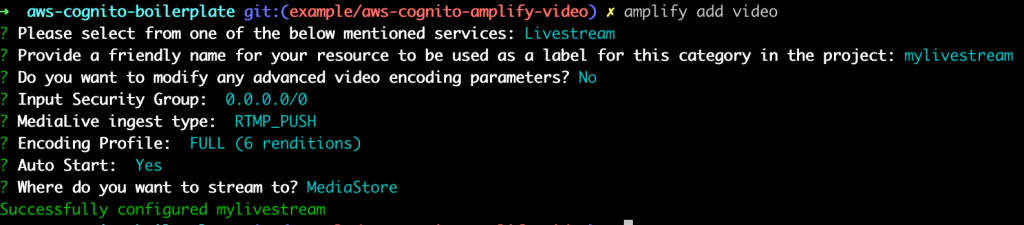
Then:amplify add video
yarn start
ornpm start
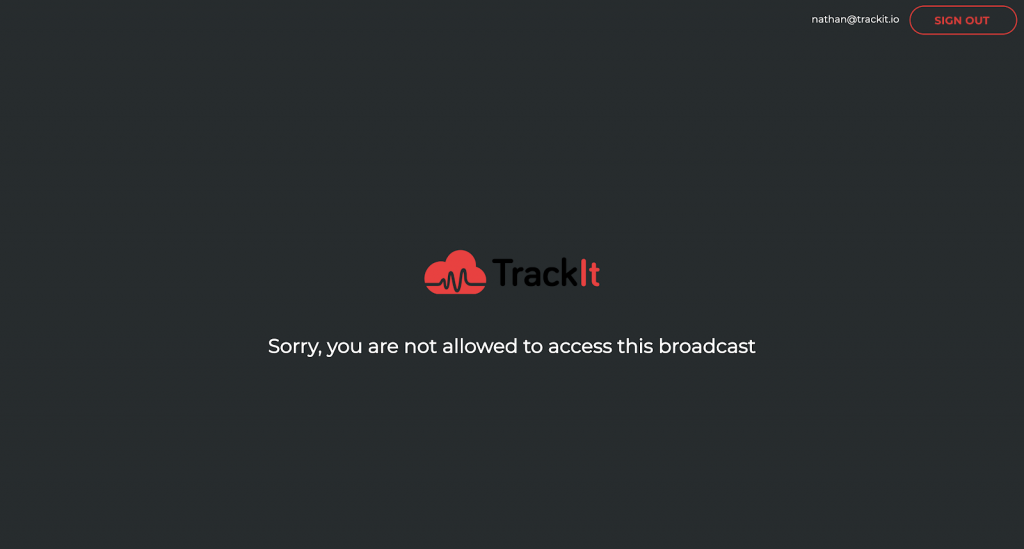
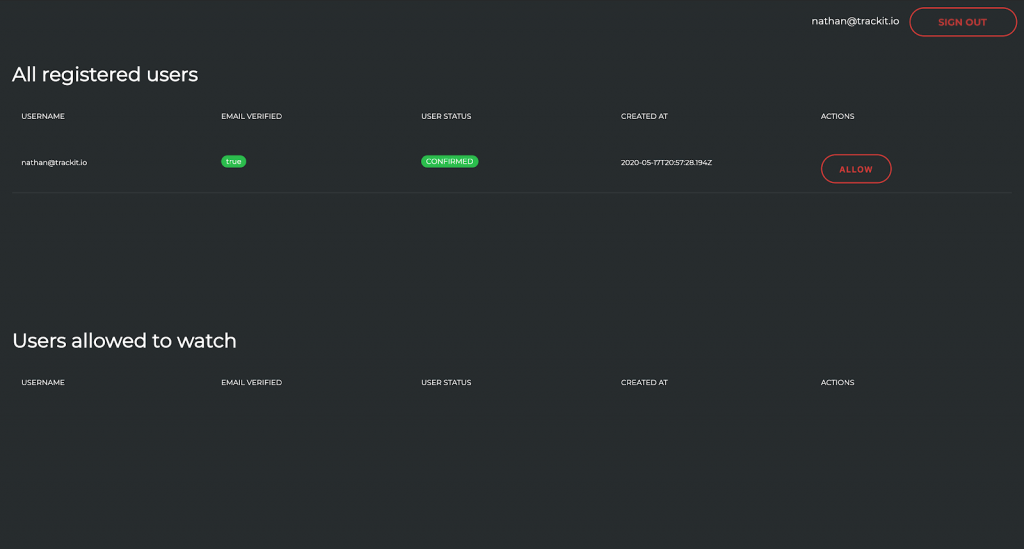
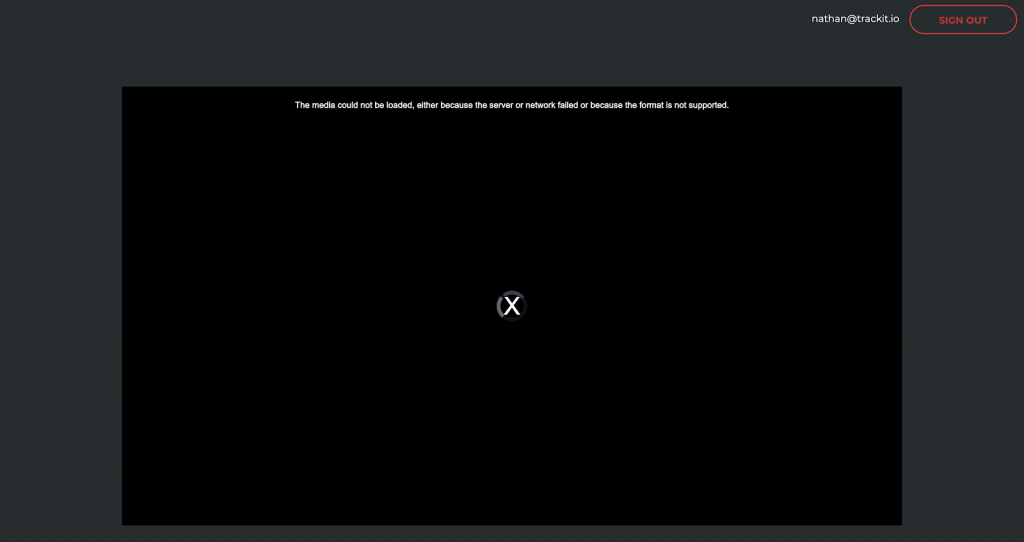
By default, because you are not part of any group, you’ll see this screen:
Users that are part of admin group will see this screen:
Standard users that are part of the user group will see this screen:
“At TrackIt, we’re always trying to automate and accelerate our delivery capabilities and this boilerplate allows us to provide solutions more rapidly and repeatedly for our customers” – Ludovic Francois, CEO of TrackIt
GitHub:
https://github.com/trackit/aws-cognito-boilerplate/tree/example/aws-cognito-amplify-video