Written by Maram Ayari, DevOps Engineer
Contents
About React
React is a JavaScript library that helps developers build user interfaces (UIs) for web pages and mobile applications. React is known for being efficient, flexible, and declarative, and is often used to develop web apps that require frequent data changes.
About Remix & NextJS
Remix is a dynamic web React framework that leverages modern web standards to deliver a robust, high-performance user experience. It enables server-side rendering, simplifying data loading, form submission, and error handling.
Next.js is a popular open-source React framework that simplifies the process of building web applications. Developed and maintained by Vercel, Next.js has gained immense popularity in the web development community due to its performance, developer-friendly features, and support for server-side rendering.
What is Server-side Rendering (SSR)?
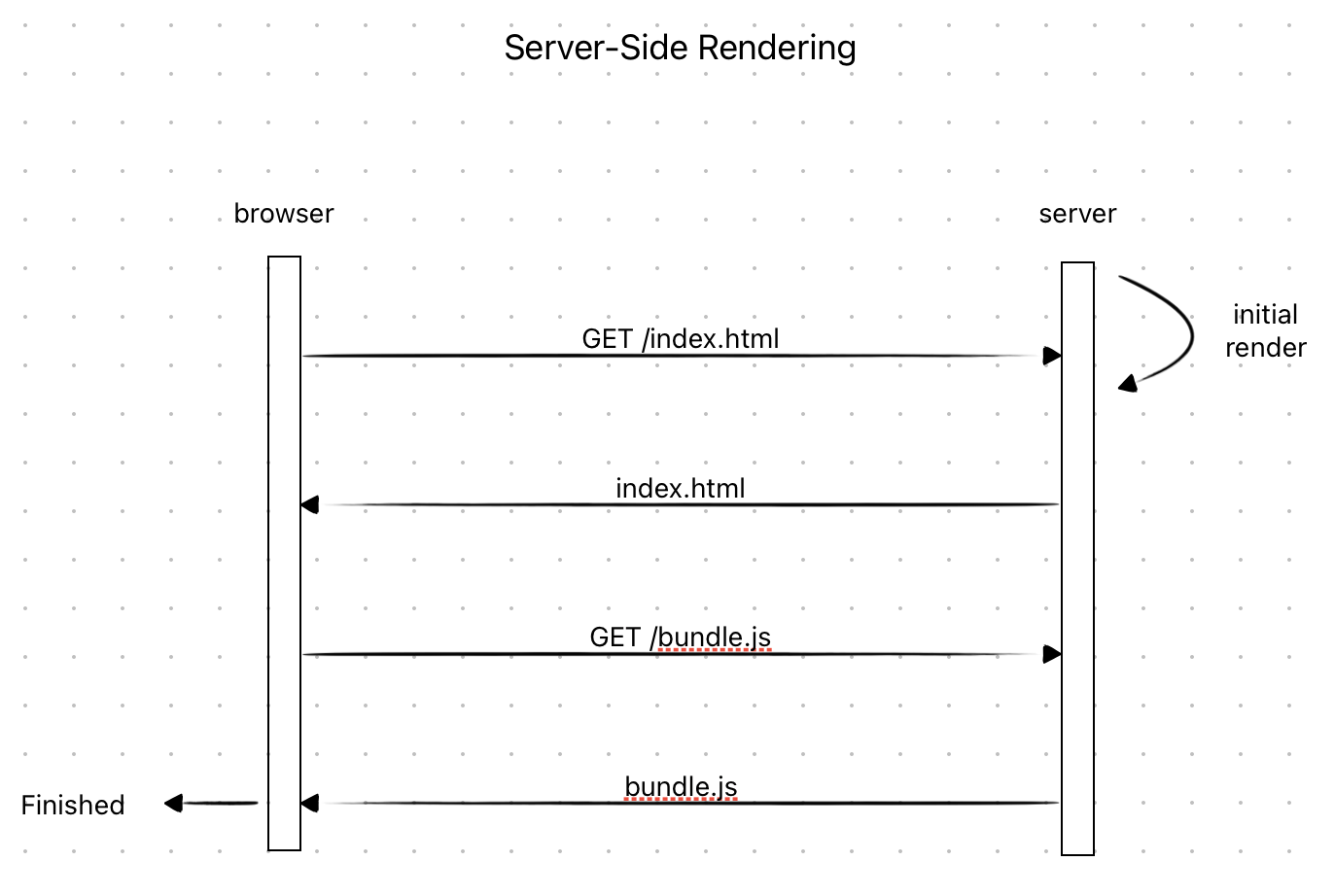
Server-side rendering known as SSR, is a web development technique in which a web page is rendered on a server before the fully rendered HTML markup is sent to the client.
SSR Process:
- User Visits URL: A user navigates to a URL in their browser.
- Server Receives Request: The server receives the request and identifies the requested route or URL.
- Data Fetching: The server retrieves necessary data from databases, external APIs, etc.
- Template Rendering: The server combines fetched data with page structure using a templating engine or framework to generate HTML markup.
- Sending Rendered Page: The server sends the fully rendered HTML page to the user’s browser as the response (not interactive).
- Displaying Page: The user’s browser displays the page without waiting for additional JavaScript execution.
- Rehydration: The server also sends the required JavaScript for client-side interactivity. This JavaScript “rehydrates” the static HTML into an interactive web app.
Benefits of Server-Side Rendering:
- Faster loading times: SSR improves initial load speed which can be important for Search Engine Optimization or SEO. It achieves this by sending fully rendered HTML to the client, eliminating the need to wait for JavaScript to render content. This enhances the user experience and improves core web vitals such as First Contentful Paint (FCP) and Largest Contentful Paint (LCP).
- Improved security: SSR reduces security risks by keeping application logic and code on the server, preventing sensitive information from being exposed to clients, and reducing the likelihood of malicious attacks.
- Richer user experience: SSR eliminates loading screens and provides a seamless browsing experience by delivering fully rendered pages to clients, increasing engagement and retention.
Limitations of Server-Side Rendering:
- Complexity: SSR applications are generally more complex because they need to handle both rendering interactive pages and leveraging the benefits of SSR, which includes sending pure HTML to the client. This process involves code-splitting and hydration. Code-splitting involves dividing the code into manageable parts. These parts are then injected or “hydrated” into the client’s page to add interactivity and functionality.
- Server load: SSR may increase the server load because it processes each user request on the server. This may cause server performance issues in heavy-traffic situations.
- Hydrations Errors: When rendering on the server, the initial HTML generated might not exactly match the structure React expects on the client. This can result in discrepancies in data fetching, component rendering, or conditional rendering.
Remix VS NextJs Comparison
1. Architecture
Remix: Utilizes a server-rendered architecture where most of the rendering work is done on the server side, providing a traditional server-rendered approach.
Next.js: Offers flexibility in rendering methods, allowing developers to choose between static generation, server-side rendering, or client-side rendering based on their application’s needs.
2. Routing
Both Next.js and Remix use a file-based routing system for their respective routes.
In Next.js, the designated routes folder is the “pages” directory found in the root directory and any file or folder created here is treated as a route.
In Remix, the designated routes folder is called “routes” and can be found in the app directory of the application. Any file or folder created here is also treated as a route.
a. Index Route
Both Remix and Next.js use index files to automatically route to a parent folder in their respective routes directory. Alternatively, routes can be based on the actual file names.
b. Nesting Route
Remix: Nesting routes is achievable using the Outlet component, which indicates where a parent route should render a nested child route. This allows the creation of a hierarchy of deeply nested routes to build complex nested layouts.
Next.js: Next.js comes with its own router and supports route nesting. To create a nested layout, the layout must be rendered on each page manually or added from the _app page with custom logic.
c. Dynamic Route
Different content can be rendered based on URL parameters using a single dynamic route file.
Remix: Created by starting the name of the dynamic route with a dollar sign (e.g., routes/post/$postid.jsx). Because Remix is based on React Router, the useParams hook can be used to access the URL parameter.
Next.js: Created using a pair of square brackets with the dynamic parameter’s name inside (e.g., pages/post/[postid].js). The useRouter hook provided by Next.js can be used to obtain the URL query parameter.
3. Data Fetching
Remix: In Remix data is fetched in the loaders. Each route can define a loader function that provides relevant data to the route when rendering. useLoaderData provides the loader’s data to the component. Loaders are only running on the server.
Next.js: Next.js supports both server-side rendering and static site generation.
- getServerSideProps: Fetches data on the server during each request. This is used for server-side rendering (SSR). Data is fetched when the page is requested by the client.
- getStaticProps: Fetches data at build time, generating static HTML pages with pre-rendered content.
- getInitialProps: Runs on both server and client, enabling data fetching for initial rendering and client-side hydration. It is a legacy API.
- fetch: Next.js extends the native fetch Web API to allow us to configure the caching and revalidating behavior for each fetch request on the server. fetch with async/await can be used in Server Components, in Route Handlers, and in Server Actions.
4. Data Mutation
Remix: Remix re-introduces HTML forms for data mutations, which can provide a more declarative and easy-to-understand approach to managing data changes. This allows the usage of HTML forms as they are. Users can also choose Remix’s Form component, which emulates HTML form behavior with JavaScript, providing faster load times and a better user experience.
Next.js: Next.js handles data mutations through API routes, SWR (“Stale-While-Revalidate”), or third-party libraries.
- On the client side: It uses React’s tools to manage data within the user’s browser. Developers can also update data in response to user actions using React’s state management features
- On the server side: Next.js allows developers to handle data changes on the server, which is useful for tasks such as updating a database. This is done through server-side code such as API routes or serverless functions.Next.js also supports real-time updates. Changes made on the server can be immediately reflected in the user interface.
5. Community and Ecosystem
Remix: Remix is a newer framework compared to Next.js. Its community and ecosystem are still growing. However, it has rapidly gained popularity due to its focus on developer experience and modern web development practices.
Next.js: Next.js has a large and active community with extensive documentation, tutorials, and plugins. It is widely adopted by companies and developers for building production-ready web applications.
Summarizing the Benefits & Limitations
Benefits of Remix
- Dynamic content: With Remix, users no longer have to choose between performance and dynamic content. By taking advantage of edge computing, applications can be both dynamic and fast.
- Faster data fetching: With nested routes, Remix can fetch data in parallel, which alleviates waterfall problems (sequential data fetching that causes delays) and greatly improves performance.
- Simpler code: No need to choose between Static Site Generation (SSG), Server-Side Rendering (SSR), or Client-Side Rendering(CSR). There is only one way to fetch data by calling a loader function. Remix significantly reduces the complexity of applications.
- More resilient apps: With Remix, links and mutations still work without JavaScript. This is beneficial because users may occasionally have a spotty connection that doesn’t allow JavaScript to load.
- Effective Error Handling: With Remix’s built-in support for mutations, error handling is easier with error boundaries and catch boundaries. Race conditions are handled automatically by the framework, such as when a user clicks a button multiple times in quick succession.
- Smaller bundle size: As mutations happen only on the server, users can reduce a large amount of code that needs to be downloaded and parsed by the browser.
- Pre-made Stacks: Remix provides pre-made stacks that allow developers to get started on a new project in seconds. These stacks come with a set of pre-configured tools and configurations, reducing setup time and allowing developers to focus on building features rather than setting up the project infrastructure.
Limitations of Remix
- Problems with not having client-side state: The absence of client-side state in Remix can lead to challenges in managing real-time updates, sharing data across routes, optimizing data fetching, and writing automated tests. While Remix provides some tools and APIs to mitigate these challenges, developers may need to carefully consider their application requirements and design patterns to address these limitations effectively.
Benefits of Next.js
- Static site generation (SSG): Next.js offers SSG, which generates static HTML files at build time. This is useful for websites with content that doesn’t change frequently, providing better performance and reduced server load.
- Feature-rich framework: Next.js offers a comprehensive set of out-of-the-box features including server-side rendering, static site generation, client-side routing, and more. This feature-rich environment reduces the need for additional libraries or frameworks, streamlining development.
Limitations of Next.js
- No built-in state management: Next.js does not have a built-in state management solution. This forces users to use a third-party library such as Redux or MobX. This can add complexity to a project and make it more difficult to maintain.
- Can be slow for dynamic routing: Next.js is optimized for static routing. If users need to use dynamic routing, they may experience slower performance.
- Limited flexibility: Next.js has its own conventions and file system-based routing, which may limit flexibility for certain advanced use cases or existing project structures.
Practical Example: Creating a Homepage
The following code blocks illustrate the creation of a simple homepage using Remix and Next.js frameworks, respectively. In both examples, a React component is utilized to render the homepage UI.
Home Page Remix Code:
// app/routes/index.tsx import { Link, useLoaderData } from ‘@remix-run/react’; import { LoaderFunction, LinksFunction, json } from ‘@remix-run/node’; interface User { id: number; name: string; username: string; email: string; } export const loader: LoaderFunction = async () => { const users = await fetch(‘https://jsonplaceholder.typicode.com/users’); return json({ users }) }; export default function Index() { const { users } = useLoaderData<typeof loader>(); return ( <div> <h1>Welcome to Remix!</h1> <ul> {users.map((user) => ( <li key={user.id}> <p>{user.name}</p> <p>{user.username}</p> <p>{user.email}</p> </li> ))} </ul> <Link to=”/about”>About</Link> </div> ); } |
In Remix, the homepage is created as a React component in the app/routes directory. The loader function allows the user to fetch data from the server. It runs only on the server side and data is fetched to the component using useLoaderData. This hook allows the user to access the data fetched by the loader function within Remix components, when the user uses the useLoaderData hook, Remix automatically provides the loader data for the current route.
Home Page NextJs Code:
//app/pages/index.tsx import Link from ‘next/link’; export async function getStaticProps() { // Fetch data for the homepage const res = await fetch(‘https://jsonplaceholder.typicode.com/users’); const users = await res.json(); return { props: { users, }, }; } export default function Home({ users }) { return ( <div> <h1>Welcome to Next.js!</h1> <ul> {users.map((user) => ( <li key={user.id}> <p>{user.name}</p> <p>{user.username}</p> <p>{user.email}</p> </li> ))} </ul> <Link href=”/about”>About</Link> </div> ); } |
In Next.js, the homepage is created as a React component inside the app/pages directory. Data retrieval is done using the getStaticProps function, which retrieves data at build time. The fetched data is then passed to the component as props.
Summary Comparison Table
Feature | Remix | Next.js |
Architecture | Server-Side Rendering | Supports Server-Side Rendering (SSR), Static Site Generation (SSG), and (Client-Side Rendering) CSR |
Routing | Flat-file-based routing | Offers both App Router and Pages Router |
Data Fetching | Loader functions on server side | getServerSideProps, getStaticProps, fetch |
Community & Ecosystem | Smaller community | Large, active community with extensive resources |
Conclusion – Making the Right Choice
Both Remix and Next.js offer robust frameworks for web development, each with its own set of strengths and considerations. For projects requiring a focus on server-side rendering, data loading, and a streamlined developer experience, Remix emerges as a compelling choice. Its emphasis on dynamic content delivery, faster data fetching, and simplified code structure makes it particularly well-suited for applications where performance and scalability are paramount.
On the other hand, Next.js presents a versatile solution, catering to a wide range of rendering methods including server-side rendering, static site generation, and client-side rendering. With its extensive ecosystem, strong community support, and out-of-the-box features, Next.js excels in scenarios where flexibility, SEO optimization, and rapid development are key priorities.
Ultimately, the choice between Remix and Next.js hinges on the specific requirements and objectives of a project. By carefully evaluating factors such as performance needs, development complexity, and long-term scalability, developers can make an informed decision that aligns with their project goals and delivers optimal results.
About TrackIt
TrackIt is an international AWS cloud consulting, systems integration, and software development firm headquartered in Marina del Rey, CA.
We have built our reputation on helping media companies architect and implement cost-effective, reliable, and scalable Media & Entertainment workflows in the cloud. These include streaming and on-demand video solutions, media asset management, and archiving, incorporating the latest AI technology to build bespoke media solutions tailored to customer requirements.
Cloud-native software development is at the foundation of what we do. We specialize in Application Modernization, Containerization, Infrastructure as Code and event-driven serverless architectures by leveraging the latest AWS services. Along with our Managed Services offerings which provide 24/7 cloud infrastructure maintenance and support, we are able to provide complete solutions for the media industry.
About Maram Ayari
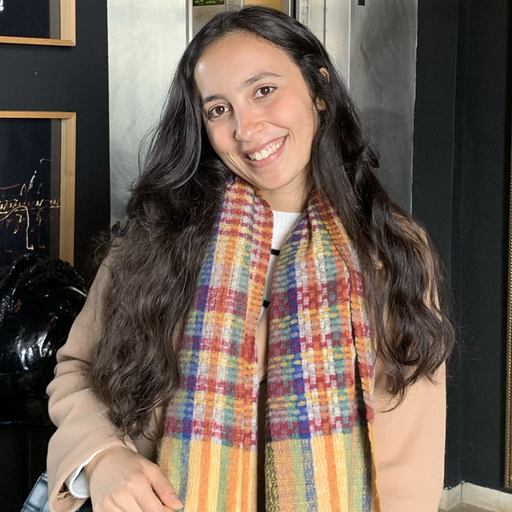
DevOps Engineer at TrackIt with almost a year of experience, Maram holds a master’s degree in software engineering and has deep expertise in a diverse range of key AWS services such as Amazon EKS, Opensearch, and SageMaker.
Maram also has a background in both front-end and back-end development. Through her articles on medium.com, Maram shares insights, experiences, and advice about AWS services. She is also a beginner violinist.