Written by Nathan de Balthasar, Software Engineer, and Timothe Coniel, Software Engineer
This project discussed below focused on modernizing an outdated video player by transitioning from a custom JavaScript library built on an older version of Video.js (v5.12.6) to a more robust solution using React and the latest version of the video.js library (v8.16.1). The upgrade was essential for incorporating the latest features and enhancements while ensuring a smoother, more engaging user experience.
By leveraging React with TypeScript, the project aimed to enhance development efficiency and maintainability.
Contents
Understanding the Legacy system
Video.js is an open-source HTML5 video player framework known for its flexibility and ability to support various video formats. In the legacy system discussed in this project, it served as the core library for video playback, but the older version presented several challenges.
The outdated version of the player became harder to maintain over time, lacking modern features and struggling with performance, especially when handling larger video files or adapting to different network conditions. Its older architecture also made it less adaptable to newer web technologies. Without regular updates, it became increasingly vulnerable to security risks. This highlighted the need for a more maintainable and up-to-date solution like React.
To overcome these limitations, it was necessary to upgrade to a newer version of Video.js and integrate it with React components. The modified solution aimed to enhance maintainability, performance, and security, ensuring a seamless and future-proof video playback experience.
Transition from Video.js v5.12.6 to v8.16.1
Upgrading the video.js library version brought several updates to enhance the video player’s capabilities and user experience. These include improved handling of streaming content through enhancements to Video Handler Session (VHS), better accessibility features, and an updated user interface with new icons for a more intuitive experience. Additionally, the codebase underwent substantial refactoring, improving maintainability and performance by modernizing dependencies and removing outdated code.
Security was also improved with updates focused on strengthening the player against vulnerabilities and phasing out support for older browsers to leverage modern web technologies.
Benefits of Moving to React
Moving to React offers significant benefits, particularly in enhancing user experience. React’s component-based architecture enables faster load times and smoother interactions, resulting in a more engaging and seamless video playback experience.
The modular approach of React simplifies development by breaking the video player into reusable components. This structure makes the code easier to manage, update, and debug, while also allowing for quicker feature implementation and more efficient troubleshooting. Storybook was employed to document and test components in isolation, ensuring consistency across the application.
The Migration Process
The migration process was planned to ensure a seamless transition from the legacy video player to a modern React-based solution. It began with an in-depth analysis of the existing system, identifying custom features and potential challenges. A detailed roadmap was created, outlining each step, from setting up the React environment to breaking down the video player into modular components. Key changes included refactoring the legacy code into React components, integrating custom features like encrypted subtitles and ad insertion, and implementing performance and security enhancements.
Basic wrapping of the video.js player in React
import React, { useEffect, useRef } from “react”; import videojs from “video.js”; import “video.js/dist/video-js.css”; interface VideoPlayerProps { src: string; width?: number; height?: number; } const VideoPlayer: React.FC<VideoPlayerProps> = ({ src, width = 640, height = 264, }) => { const videoRef = useRef<HTMLVideoElement>(null); useEffect(() => { if (videoRef?.current) { const player = videojs(videoRef.current, { controls: true, autoplay: false, preload: “auto”, }); return () => { player.dispose(); }; } }, [videoRef]); return ( <div data-vjs-player> <video ref={videoRef} className=”video-js” controls preload=”auto” width={width} height={height} > <source src={src} type=”video/mp4″ /> </video> </div> ); }; |
Storybook was used to document and test components individually. Integration tests ensured all components worked together smoothly in the new framework using Vitest. The careful planning and execution resulted in a final product that not only preserved but improved upon the original system’s functionality and reliability.
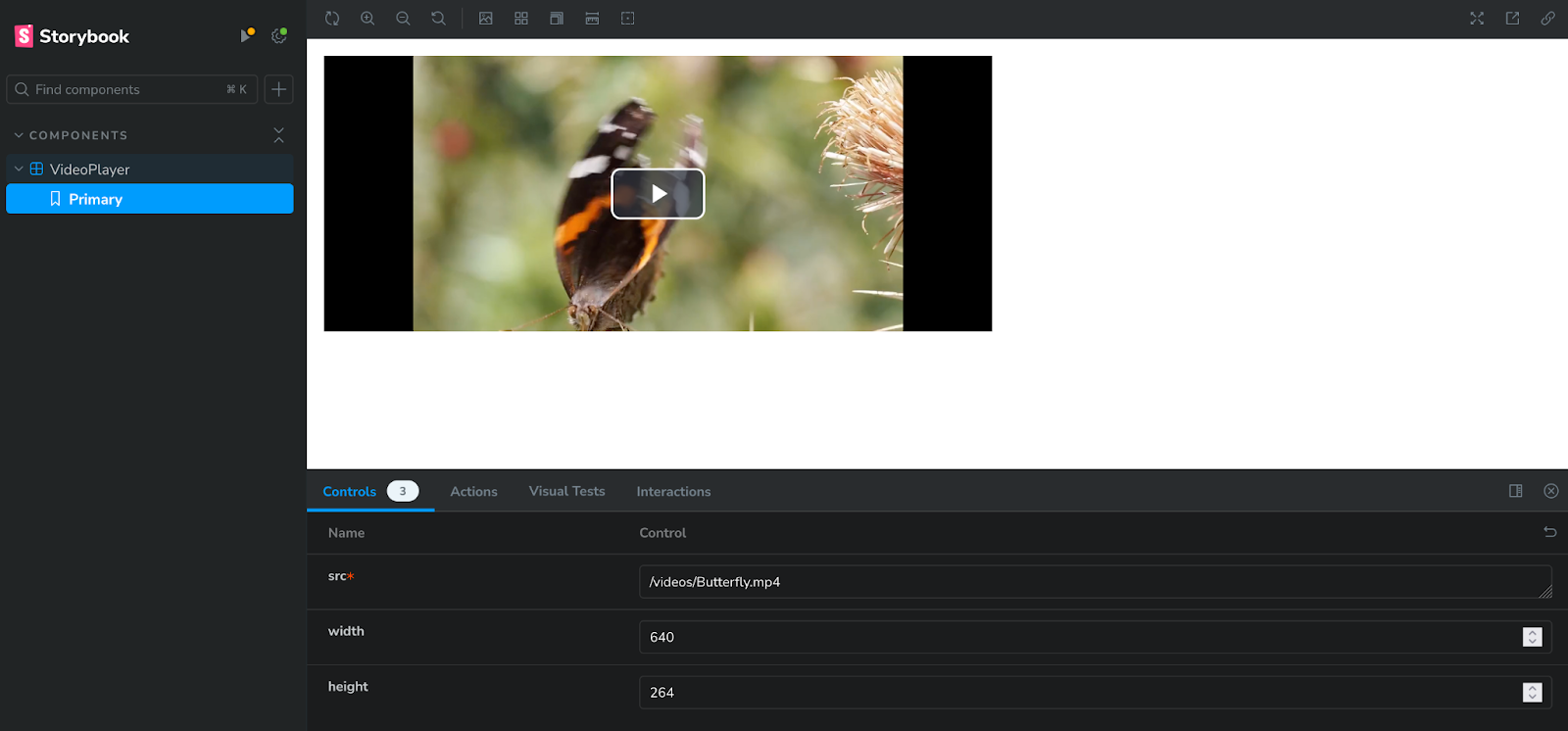
Overcoming Challenges and the Outcomes of the Upgrade
Upgrading a legacy system comes with its share of challenges, both technical and non-technical. Common obstacles include dealing with outdated code that is difficult to maintain and is lacking compatibility with modern technologies. Additionally, integrating custom features such as encrypted subtitles and ad insertion into the new framework requires careful planning and execution.
The structured approach employed by the development team i.e. refactoring the legacy code into modular React components and using tools like Storybook for isolated testing helped address these challenges. This not only simplified the development process but also ensured that each feature was seamlessly integrated into the new system.
Ensuring a smooth transition for end users was another critical focus. The upgrade was designed to be as seamless as possible, with minimal disruption to the user experience. Improved performance, faster load times, and a more responsive interface were immediate benefits, enhancing overall user satisfaction. React’s architecture also future-proofed the application by making it easier to update and extend in the future.
The upgrade had positive outcomes for both the development team and users. Developers benefited from a more maintainable and scalable codebase, while users enjoyed a more modern, reliable, and engaging video playback experience. This strategic upgrade not only resolved existing issues but also laid the groundwork for easier updates and positioned the application for long-term success.
Closing Thoughts
The project successfully modernized a legacy video player by transitioning to React and upgrading to a newer version of Video.js. This migration not only improved performance and user experience but also made the system more maintainable and scalable for future updates. Careful planning and execution ensured a seamless transition, preserving the essential custom features while enhancing the overall functionality.
Keeping up with technology upgrades can be a crucial for modern day infrastructures that are constantly in a state of evolution. Regular updates ensure that applications remain secure, compatible with new technologies, and capable of delivering the best possible user experience. This project highlights the importance of proactive upgrades and adopting modern development practices to future-proof applications.
Looking ahead, media application development will continue to evolve with advancements in frameworks and technologies. By embracing these changes and maintaining a flexible, forward-thinking approach, developers can create robust, high-performing media solutions that meet the demands of users and the industry. This project is a testament to the value of staying current with technology trends, setting the stage for continued innovation and success in the future.ile and responsive in today’s fast-paced environment.
About TrackIt
TrackIt is an international AWS cloud consulting, systems integration, and software development firm headquartered in Marina del Rey, CA.
We have built our reputation on helping media companies architect and implement cost-effective, reliable, and scalable Media & Entertainment workflows in the cloud. These include streaming and on-demand video solutions, media asset management, and archiving, incorporating the latest AI technology to build bespoke media solutions tailored to customer requirements.
Cloud-native software development is at the foundation of what we do. We specialize in Application Modernization, Containerization, Infrastructure as Code and event-driven serverless architectures by leveraging the latest AWS services. Along with our Managed Services offerings which provide 24/7 cloud infrastructure maintenance and support, we are able to provide complete solutions for the media industry.
About Nathan De Balthasar
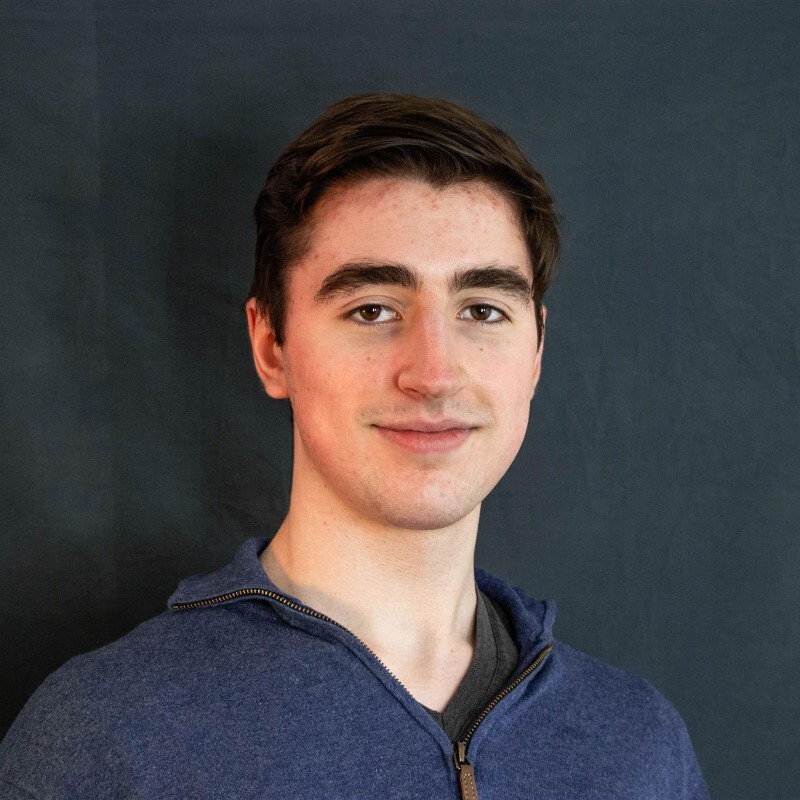
Software Engineer at TrackIt for over a year, Nathan specializes in Infrastructure as Code (IaC), serverless workflows, and event-driven architectures.
His expertise in designing and implementing automated systems and scalable cloud solutions streamlines operations and enhances system reliability and performance.
About Timothe Coniel
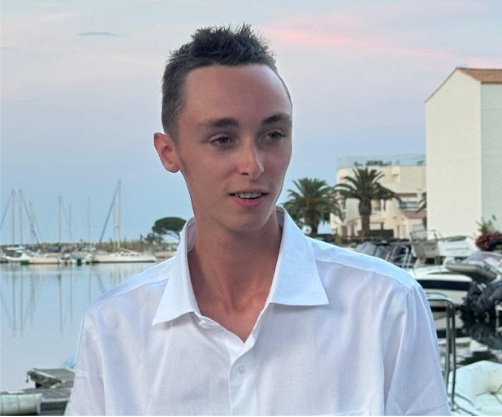
Timothe is a junior software engineer at TrackIt. With a few months of experience at the company, he is specializing in AWS services and developing scalable media solutions. He is passionate about mobile applications and web development.