AWS Amplify and Amplify Video is a fast and simple way to create a video streaming site., It’s often necessary, however, for administrators to have an effective means to manage their media content.
In this tutorial, readers will learn how to create a simple media asset management panel and link it to an Amplify-based back end.
To create the panel, we use the react-admin module, which is a front-end framework for building data-driven applications that will run in a typical web browser.
Prerequisites
- A working React project
- Some GraphQL requests already prepared to communicate with the Amplify based back end
The first step is to install react-admin on the project. Use one of the 2 following commands (depending on the package manager being used):
npm install react-admin |
Or
yarn add react-admin |
Admin Component
The next step is to create a route ready to host the admin page. If using a react-router, create a route by adding a <Route /> between the switch tags as seen in the snippet below:
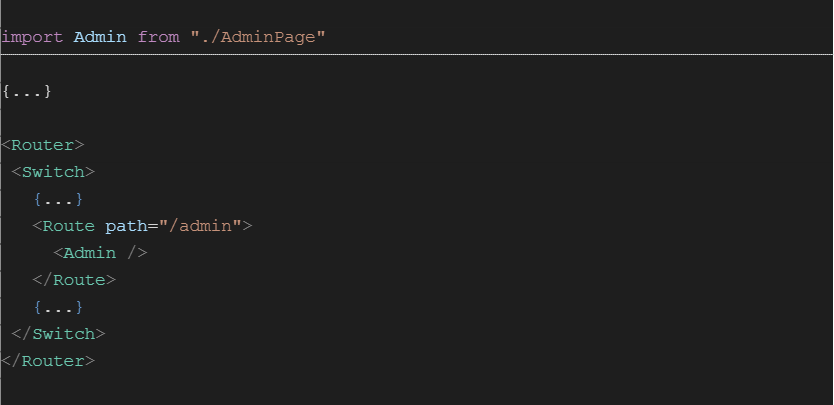
Once the route is ready, create the following Admin component:
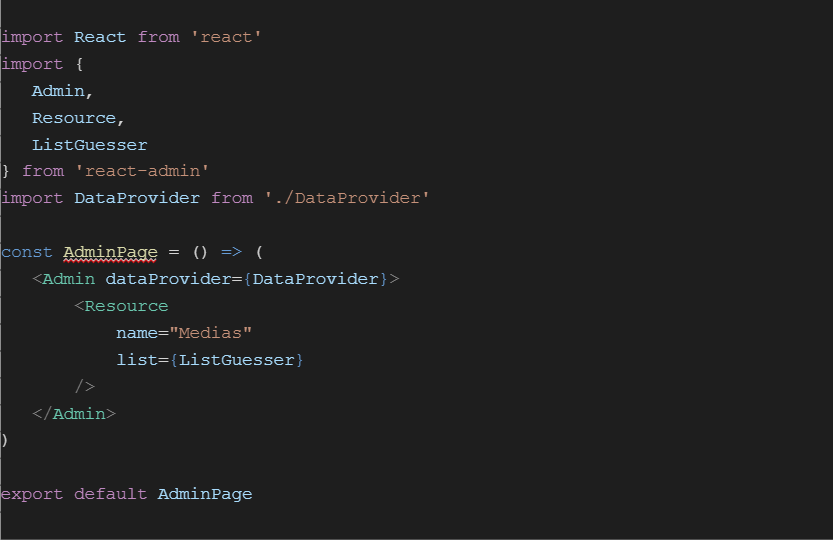
There are 3 important components in this code snippet:
DataProvider:
The component that is used as a link between react-admin and the back end. It has to be declared in a specific format as discussed later in the article.Resource
: Each model being managed should have its own resource. In this case, the resource represents the media. For each of these resources, there is an option to create, edit, list, and delete one or more assets. The name is very important here so it is suggested to replace “Medias” with the name of the resource (videos, live, etc.).ListGuesser
: A very powerful react-admin component, ListGuesser analyzes the data and creates a table with a default column setup. All the assets are listed in this table. This component is temporary and should be modified later to match user requirements.
DataProvider
As mentioned earlier, DataProvider is an object used to create a link between GraphQL and the react-admin module. Depending on the page users are on, react-admin calls one of the following functions of DataProvider:
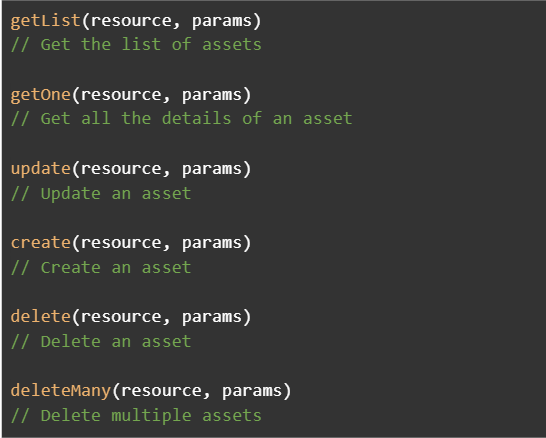
DataProvider Functions
Each of these functions must return a Promise (the eventual result or value of an operation). For the moment, list
is the only property defined for the <Resource>
component. The next step is to create the function getList that fetches the list of media assets:
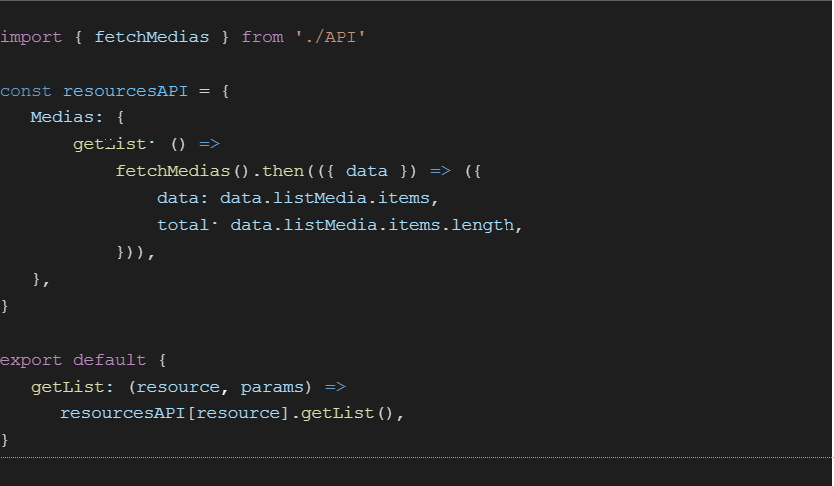
The resource
parameter will receive the value “Medias” since the property name
of the <Resource> has been defined as “Medias”.
In the example presented in the code snippet above, the function fetchMedias()
has been used. Users have to type in the appropriate function instead of fetchMedias()
to send the GraphQL query that fetches the list of all the media assets. As mentioned earlier, this function has to return a Promise.
As seen in the code snippet above, a map of resources is used to link and facilitate queries. Companies managing multiple resources should consider a similar strategy.
In the .then(({ data })
function used in the code snippet above, the data is formatted to ensure that the getList: ()
function returns data in the following format:
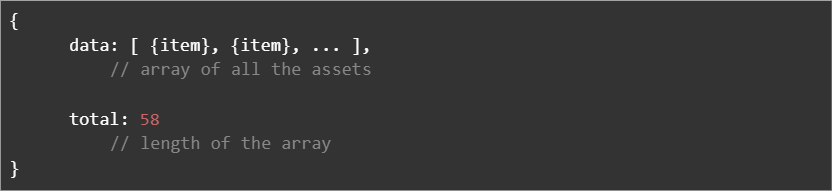
Data Formatting Example
The function parameter params
could also be used to filter the list in the future.
What follows is the result of the admin code written above when the React app is launched using the appropriate launch command, yarn start
or npm run start
for example.
Screenshot of the Admin Panel
List Component
From the admin panel’s browser tab, open the Javascript console. It should display code similar to what’s in the screenshot below:
List Component Generated By ListGuesser
ListGuesser should have generated the code to create the list component of the Media resource.
Copy this code and paste it inside a new Javascript file. Next, in the AdminPage.js file, import the newly created component and replace ListGuesser with this component. Next, delete the columns that are not needed (for example, the ‘id’ column):
MediasList.js
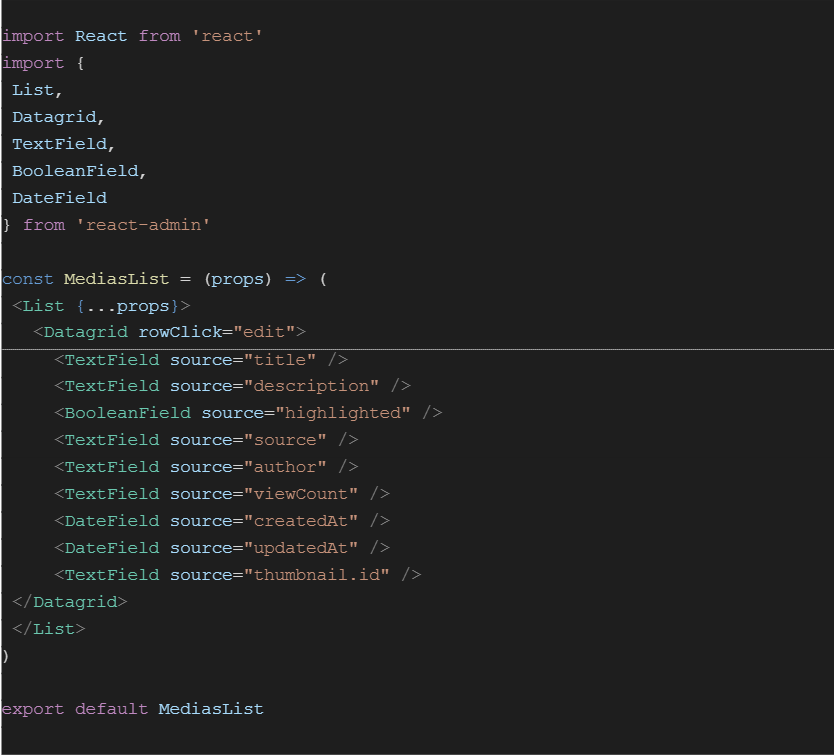
Replace ListGuesser:
AdminPage.js
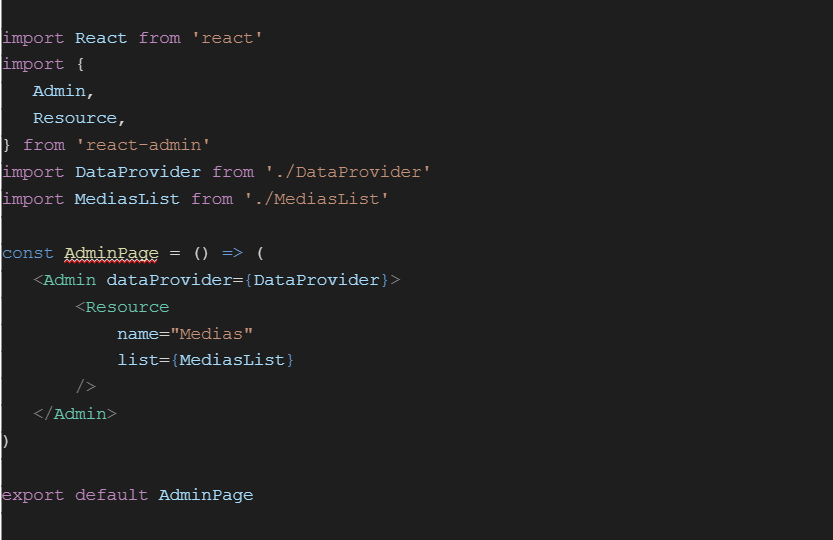
Custom Fields
A list of all available fields can be found in the react-admin documentation. Oftentimes, users choose to implement a Custom Field to display the data exactly as required.
In this example, a field called “source” in the Media model can only take one of two string values – “YOUTUBE” or “SELF”. Instead of displaying one of these 2 strings in a TextField, create a custom field that displays the Youtube icon or the Amplify icon
.
Create a file named SourceField.js (the icons have already been prepared and placed in a folder named ‘assets’):
SourceField.js
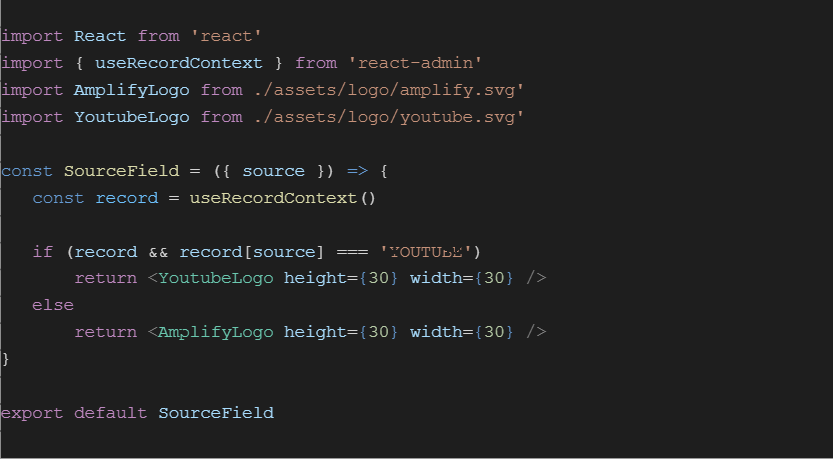
SourceField Component Code Snippet
The hook useRecordContext is used to retrieve the asset being rendered, and access the correct property of this asset using the source
prop. This prop contains the string “source” but it could also contain the string “thumbnail” if a custom field for thumbnails is required.
Next, replace the TextField in our file MediasList.js:
MediasList.js
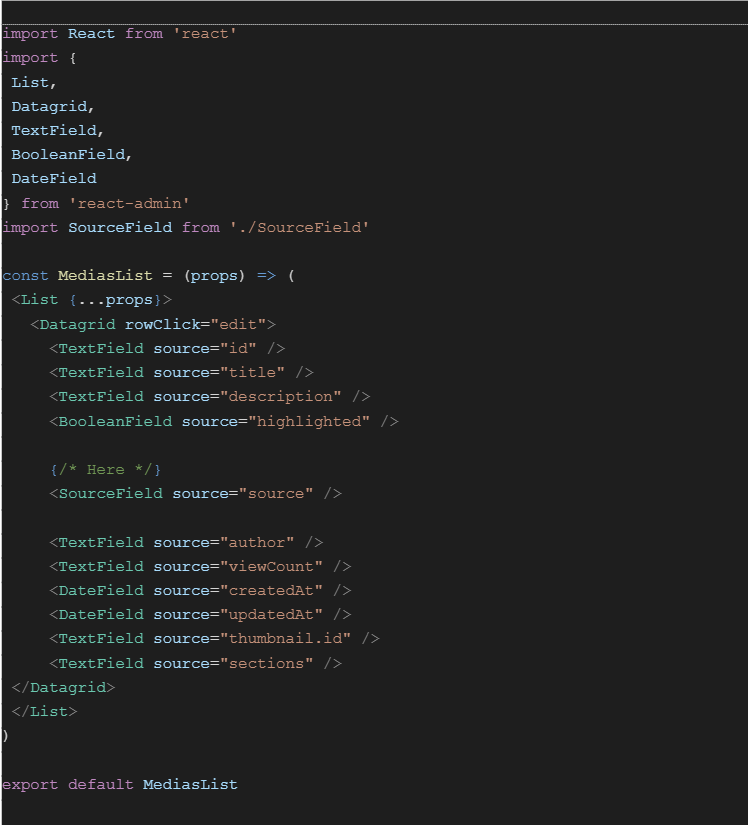
TextField Replaced With SourceField
Next, test the new field in the internet browser:
Before Adding SourceField vs. After Adding SourceField
Create, Edit, and Delete
To implement the create, edit, and delete features, first fill in DataProvider with all the missing queries:
DataProvider.js
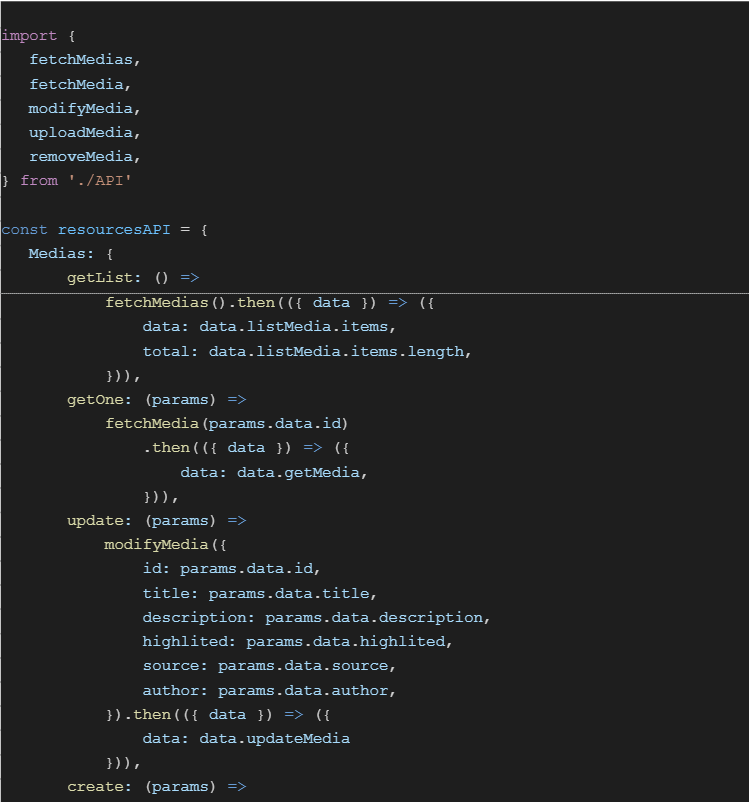
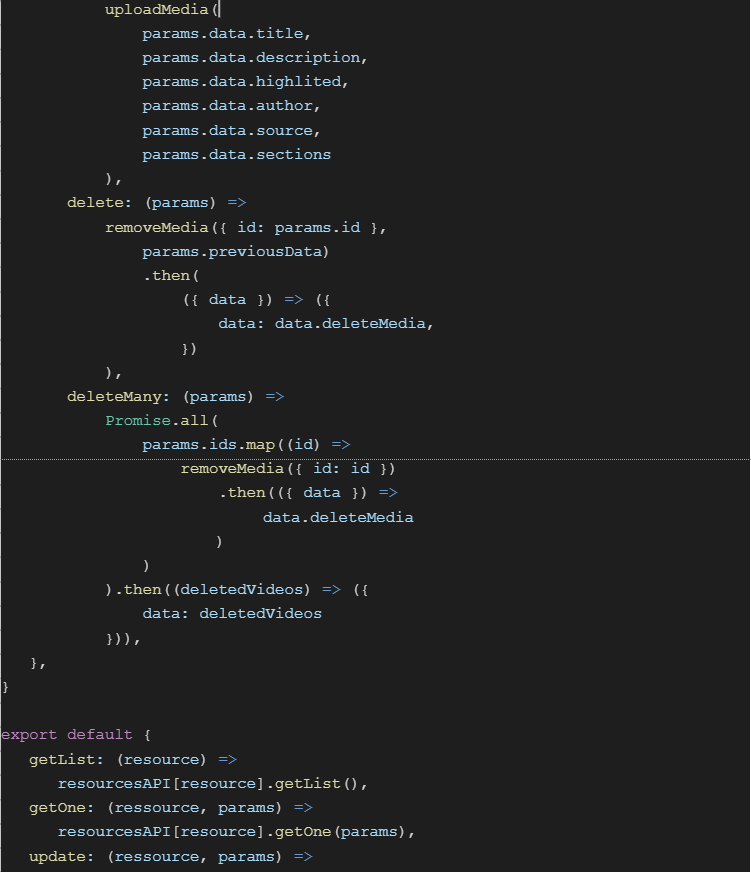
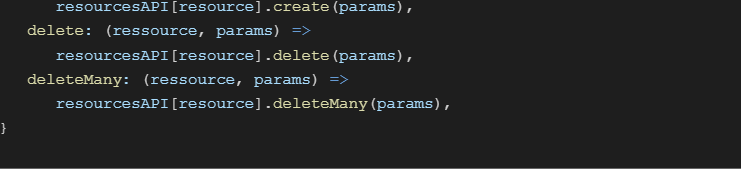
Filling In DataProvider Queries
All the functions seen here return Promises that will resolve with an object containing property data. Readers have to carefully format the objects returned by all the functions included in DataProvider.
For example, in the case of the function deleteMany, format the object returned by the Promise in the following way:
{ |
Example of an Object Returned by the Promise
Finally, follow the same logic as earlier to create the MediaEdit.js using EditGuesser.
AdminPage.js
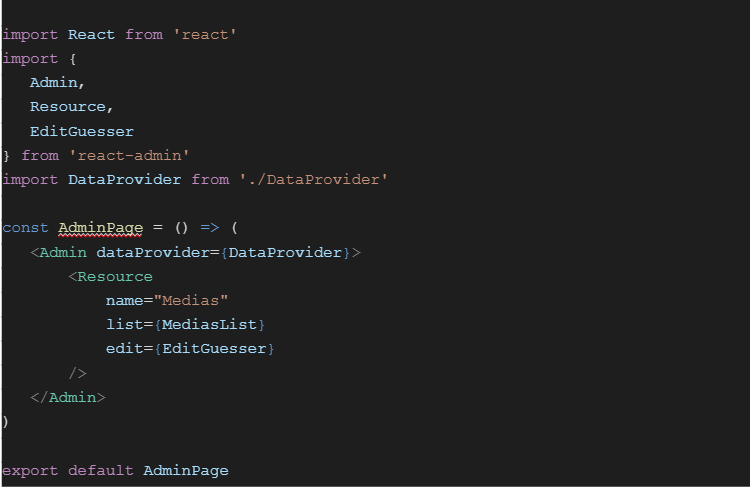
Edit Feature Implemented Using EditGuesser
In the browser, when we click on an asset in the list, we find the Edit component code that react-admin generated in the console:
Edit Component Generated By EditGuesser
Once again, copy and paste this into a new file and replace EditGuesser with the new Edit component. Then proceed to delete all the inputs no longer required. In addition, readers could also create custom inputs similar to what was done for the custom fields (see documentation).
Next, create a CreateMedia component that is exactly the same as EditMedia with just a change in the component’s name. Then update <Resource>:
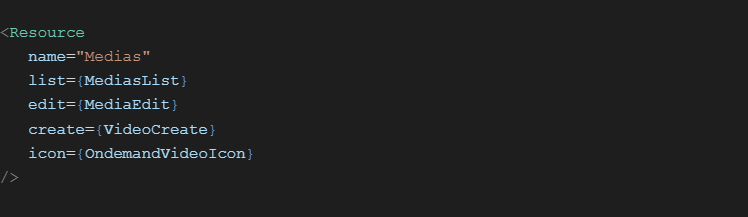
Replacing EditGuesser by MediaEdit, adding the VideoCreate Component and Adding an Icon to the Resource
Note: Readers can add icons to the menu like in the example above using the “icon” property.
Once all these steps are complete, a delete button, create button, and the edit view should be available when we click on an asset in the admin panel list:
Create Button in the Admin Panel
Delete Button in the Admin Panel
Admin Panel View when Editing an Asset
Conclusion
This article walks readers through the process of creating a simple and effective media asset management panel for an Amplify-based VOD website. TrackIt strongly recommends that readers contact TrackIt, or visit the react-admin documentation to implement more advanced features and to learn more about the full capabilities of the react-admin package.
About TrackIt
TrackIt is an international AWS cloud consulting, systems integration, and software development firm headquartered in Marina del Rey, CA.
We have built our reputation on helping media companies architect and implement cost-effective, reliable, and scalable Media & Entertainment workflows in the cloud. These include streaming and on-demand video solutions, media asset management, and archiving, incorporating the latest AI technology to build bespoke media solutions tailored to customer requirements.
Cloud-native software development is at the foundation of what we do. We specialize in Application Modernization, Containerization, Infrastructure as Code and event-driven serverless architectures by leveraging the latest AWS services. Along with our Managed Services offerings which provide 24/7 cloud infrastructure maintenance and support, we are able to provide complete solutions for the media industry.